Creating a screen and mouse jiggler with Python
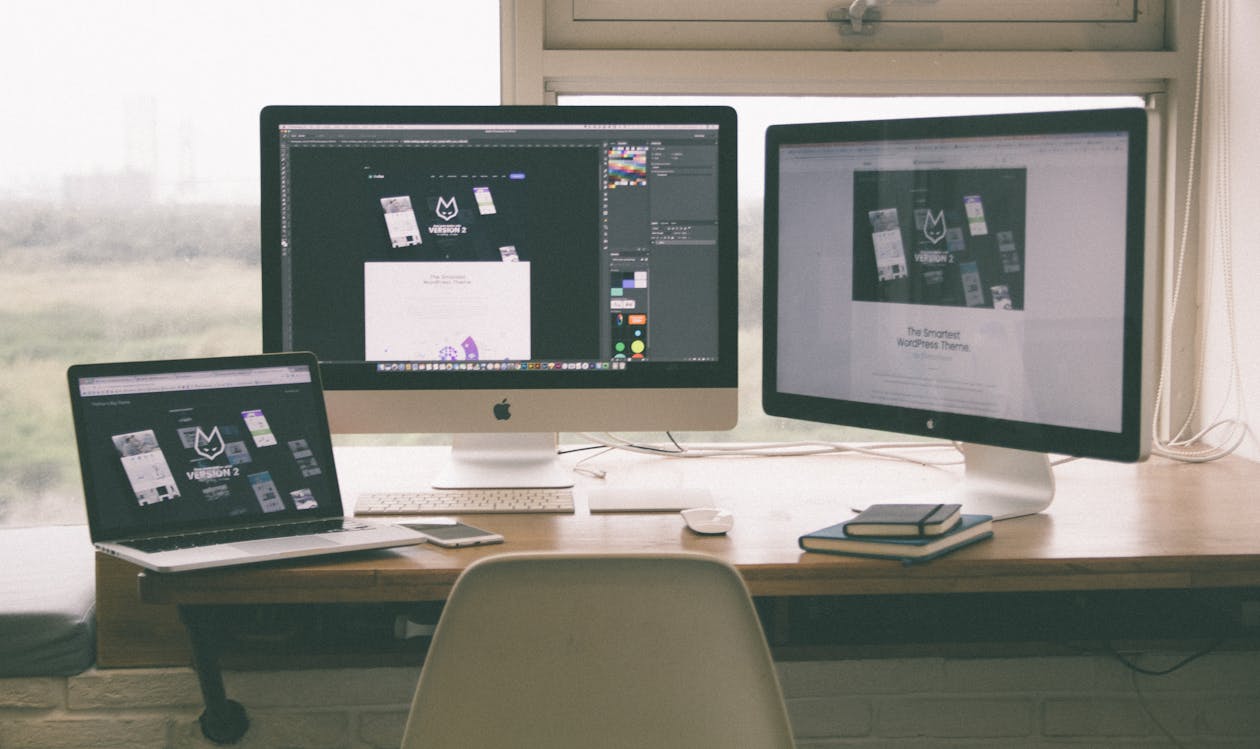
I recently came across the idea of a mouse jiggler (keeps your mouse moving) and after some investigation realised there are products being sold to achieve this! Yes, even after doing up to 50% of my time working from home since long before the COVID-19 pandemic I had never heard of this 😄 The more I thought about it, I figured something like this would be really useful for me for a number of good reasons.
Why build a mouse jiggler?
Sometimes I use my personal PC or laptop to try out ideas or perform testing outside of the organisation's internal network. However, if I spend more then approximately 1 minute away from my work laptop the screen will go off, my status will appear as 'away' on instant messenger. This makes it seem like I'm not available for my team's questions when really I'm just doing work on my own machine. I'd prefer the screen to just stay on instead using the touch pad to keep the screen on. Unfortunately, the screen saver / screen off / IM settings are disabled.
The solution could be just moving the mouse back and forth slowly on the screen to keep the active window showing, or with a function to switch windows from time to time so I can check different apps as I work.
I guess some other reasons might be balancing work and life more generally - attending appointments, having a coffee break, making lunch, or letting the dog out.
I have no doubt some people may use such a tool to avoid work and appear present at their machine, but then that's not really a mouse jiggler problem, that's a job satisfaction, productivity, motivation, wellbeing and management problem.
More generally, automation skills with Python are very good to have, and can be used in other projects like if you wanted to record your mouse and keyboard clicks to then automate repetitive tasks.
Explaining the mouse jiggler program
The only package that this program relies on is PyAutoGUI. To install with pip, run:
pip install pyautogui
Once installed create a Python file.
# -*- coding: utf-8 -*-
import pyautogui
import time
import random
import sys
pyautogui.FAILSAFE = False
def switch_screens() -> None:
"""
Switches the active screen using Alt + Tab
a random number of times.
"""
max_switches = random.randint(1, 5)
pyautogui.keyDown('alt')
for _ in range(1, max_switches):
pyautogui.press('tab')
pyautogui.keyUp('alt')
def wiggle_mouse() -> None:
"""
Wiggles the mouse between two coordinates.
"""
max_wiggles = random.randint(4, 9)
for _ in range(1, max_wiggles):
coords = get_random_coords()
pyautogui.moveTo(
x=coords[0],
y=coords[1],
duration=5
)
time.sleep(10)
def get_random_coords() -> []:
"""
Returns a list of coordinates in the
format [x=1980, y=1080]
"""
screen = pyautogui.size()
width = screen[0]
height = screen[1]
return [
random.randint(100, width - 200),
random.randint(100, height - 200)
]
if __name__ == "__main__":
print('Press Ctrl-C to quit.')
try:
while True:
switch_screens()
wiggle_mouse()
sys.stdout.flush()
except KeyboardInterrupt:
print("\n")
To start the program use this command from the same directory:
python mouse_jiggler.py
To end the program use Ctrl + C.
So the program relies on two functions:
switch_screens
uses Alt + Tab to switch the active screen a set number of times.wiggle_mouse
moves the mouse to a random set of coordinates.
These functions are using some of the many methods that PyAutoGUI conveniently provides:
.size()
returns current screen resolution width and height.moveTo(x, y, duration)
moves the mouse to XY coordinates over duration in seconds.keyDown(key)
presses the key down and keeps the button pressed.keyUp(key)
releases a key that was kept pressed bykeyDown()
.keyPress(key)
presses the given key and combineskeyDown()
followed bykeyUp()
This creates a simple solution to always keep the screen active, preventing it from turning off and keeping you appearing as online. This has worked great and really has taken a burden off my mind whilst I try to innovate and prove techniques on my own personal machine that might not work on my work machine. It really is a win-win. If you only want the mouse to move and to keep the active window showing and don't want to switch screens, remove the switch_screens function call underneath while True
.
I have heard stories of some employers using screen and keyboard tracking software for monitoring employees which I find really sad. I'm focused and I take pride in my work but I'm not always at 100% so I doubt any monitoring software would be a true reflection on how much productivity I give and how much value I bring to my workplace in terms of money and time. No one can be switched on all the time, and we all have to realise that mental health and wellbeing in general is so important. If you did find yourself in that situation and had to stick around a while and had the ability to install Python, I can see an modified version of this program being useful to spread the time between screen switches out.
You might have noticed I set FAILSAFE to false to turn it off. This is NOT recommended in the documentation so consider yourself warned, however I found to reliably avoid the failsafe action when the mouse is in any of the four corners of the primary monitor, it was best to disable it. It just means you have to be extra careful with the code, and if in any doubt set it to true to re-enable it.
Seeing the mouse jiggler in action
Here is a quick video of how the program behaves moving the mouse and switching screens a number of times.
What will you use yours for?
Okay this was a fun article, now you know how to create a screen and mouse jiggler with Python, and have a solid start to building more advanced robotic process automation (RPA) solutions with PyAutoGUI. You can refer to the documentation for more guidance on using PyAutoGUI and think about what else you might like to build 😄
If you enjoyed this article be sure to check out other articles on the site, some which also explore automation with Python and PyAutoGUI including:
- Record mouse and keyboard for automation scripts with Python
- Reduce Material Design Icons Font to 7KB and automate with PyAutoGUI
- Developing your data science and analytical coding skills - a review of DataCamp for improving your Python skills
Finally, if you have any questions or if you decide to use or extend this program, please leave a comment below. I'd love to know what you use it for and how it's helped you out 👍